Target
From MSX Game Library
(Redirected from Targets)
The choice of the target determines the final format of the game: ROM, disk, etc.
It is a parameter used by the Build Tool to adapt your code to the target during the construction of the final program and to assemble the data to be compatible with the target.
The choice of the format is made in the project configuration file (project_config.js).
Here is a description of the different target formats available in MSXgl.
Contents
Basic program

Binary program to be executed from the BASIC environment using bload function.
Target | Description |
---|---|
BIN_DISK | BASIC binary program (starting at 8000h) on disk |
BIN_TAPE | BASIC binary program (starting at 8000h) on tape |
BIN_USR | BASIC USR binary driver (starting at C000h) |
BIN | Alias for BIN_DISK |
BAS | Alias for BIN_DISK |
USR | Alias for BIN_USR |
Note: The maximum size of a BASIC program is about 24 KB (including variables).
The Build tool deployment step places your final program in several formats:
- .bin format in emul/bin/ folder
- .dsk format in emul/dsk/ folder (image of a floppy disk containing your program)
- .cas format in emul/cas/ folder (image of a tape cassette containing your program)
MSX-DOS program

Binary program to be executed from the MSX-DOS environment.
Target | Description |
---|---|
DOS1 | MSX-DOS 1 program (starting at 0100h) |
DOS2 | MSX-DOS 2 program (starting at 0100h) |
DOS0 | Direct program boot from disk (starting at 0100h) |
DOS | Alias for DOS1 |
BOOT | Alias for DOS0 |
Note:
- The maximum size of a MSX-DOS program is about 53 KB (including variables).
- For "DOS0" target, maximum is 48 KB (excluding variables).
The Build tool deployment step places your final program in two formats:
- .com format in emul/dosX/ folder (dos1, dos2 ou dos0)
- .dsk format in emul/dsk/ folder (image of a disk containing your program)
Plain ROM program
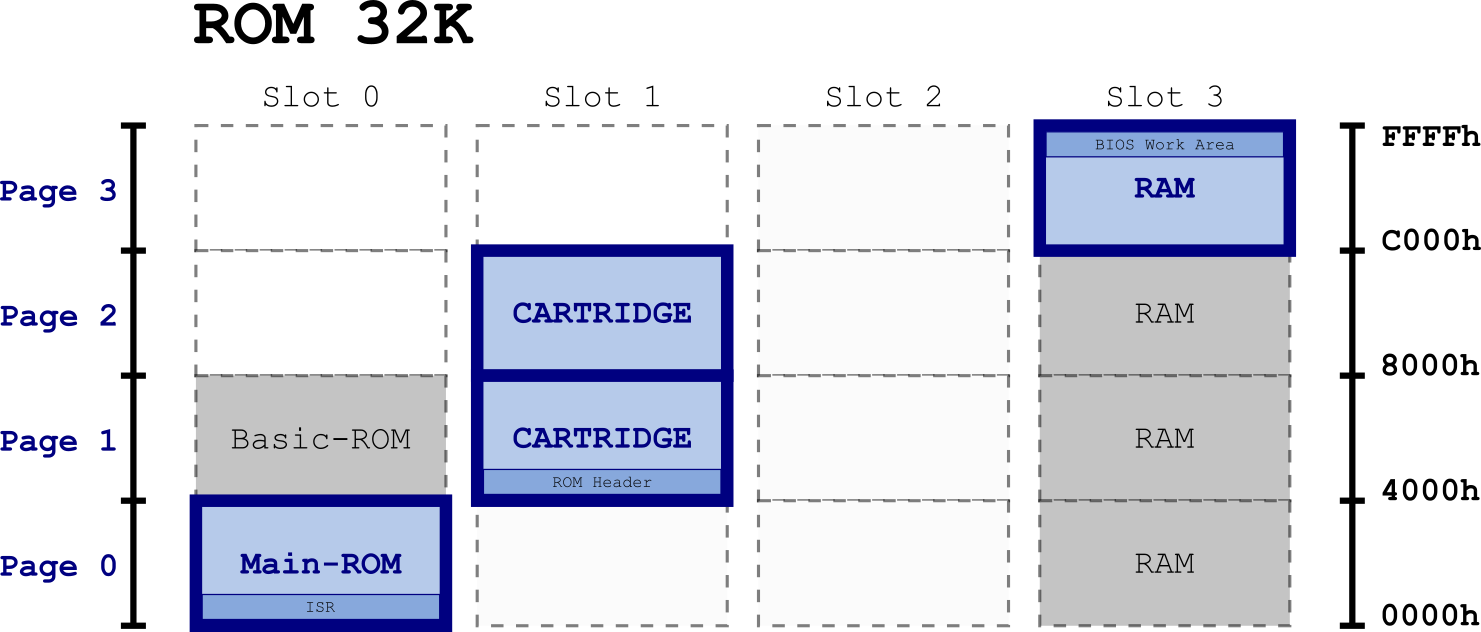
Binary program to be written into a ROM from 8 to 64 KB and executed from a cartridge.
Target | Description |
---|---|
ROM_8K | 8 KB ROM in page 1 (4000h ~ 5FFFh) |
ROM_8K_P2 | 8 KB ROM in page 2 (8000h ~ 9FFFh) |
ROM_16K | 16 KB ROM in page 1 (4000h ~ 7FFFh) |
ROM_16K_P2 | 16 KB ROM in page 2 (8000h ~ BFFFh) |
ROM_32K | 32 KB ROM in page 1&2 (4000h ~ BFFFh) |
ROM_48K | 48 KB ROM in page 0-2 (0000h ~ BFFFh) |
ROM_48K_ISR | 48 KB ROM in page 0-2 (0000h ~ BFFFh) with ISR replacement |
ROM_64K | 64 KB ROM in page 0-3 (0000h ~ FFFFh) |
ROM_64K_ISR | 64 KB ROM in page 0-3 (0000h ~ FFFFh) with ISR replacement |
ROM | Alias for ROM_32K |
See also: Create a plain ROM.
The Build tool deployment step places your final program in emul/rom/ folder.
Mapped ROM program
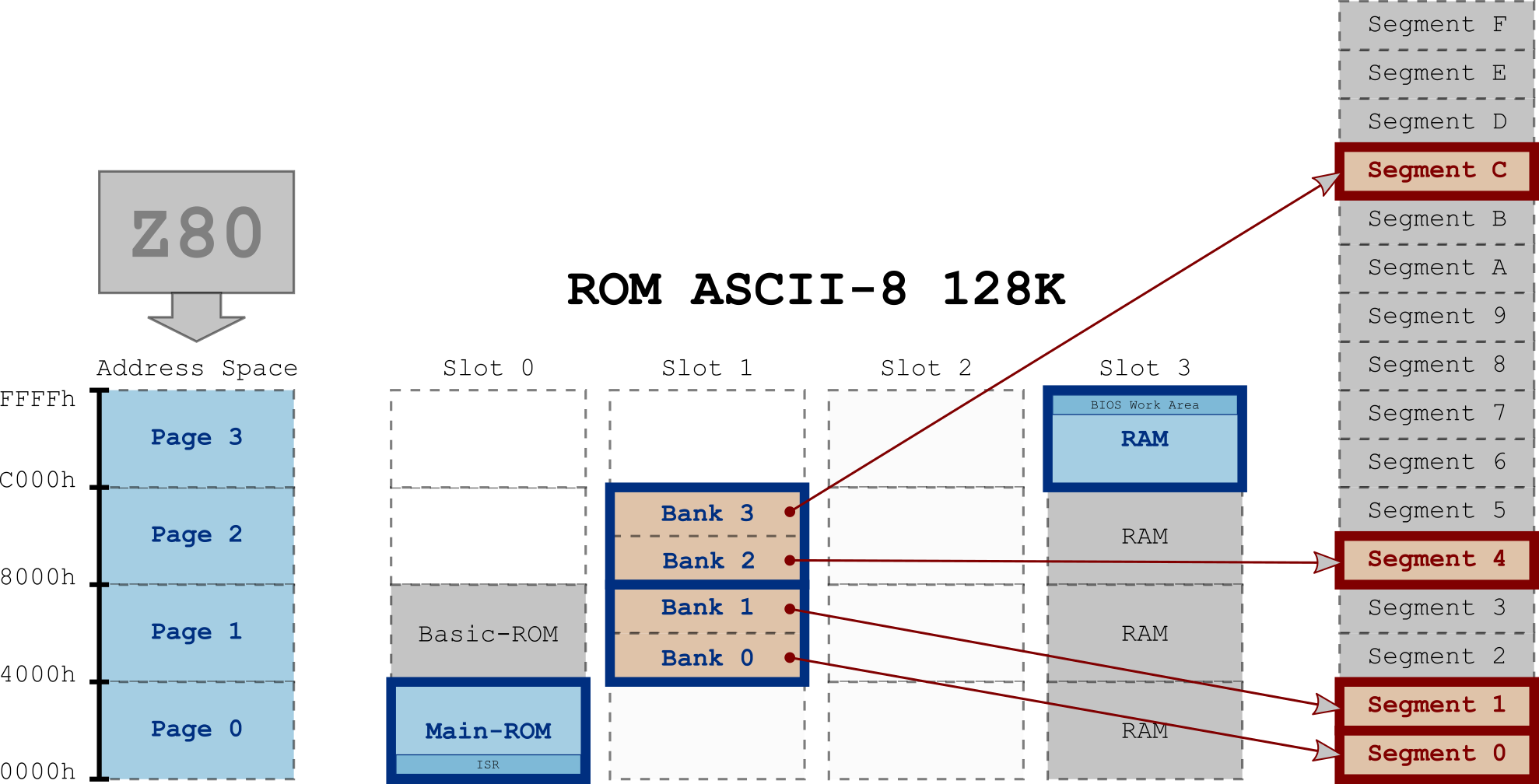
Note: "MegaROM" refers to a ROM of 128 KB or more. Even though they are not widely supported, there is nothing to prevent you from creating a 64 KB ROM using a mapper. We therefore prefer to use the term Mapped ROM here rather than MegaROM.
You can create program using one of the ROM mappers supported by MSXgl:
Target | Description |
---|---|
ROM_ASCII8 | ASCII-8: 8 KB segments for a total of 64 KB to 2 MB |
ROM_ASCII16 | ASCII-16: 16 KB segments for a total of 64 KB to 4 MB |
ROM_KONAMI | Konami MegaROM (aka Konami4): 8 KB segments for a total of 64 KB to 2 MB |
ROM_KONAMI_SCC | Konami MegaROM SCC (aka Konami5): 8 KB segments for a total of 64 KB to 2 MB |
ROM_NEO8 | NEO mapper: 8 KB segments for a total up to 32 MB |
ROM_NEO16 | NEO mapper: 16 KB segments for a total up to 64 MB |
ROM_YAMANOOTO | Yamanooto: 8 KB segments for a total of 2 or 8 MB |
ROM_ASCII16X | ASCII16-X: 16 KB segments for a total up to 8 MB |
ROM_K4 | Alias for ROM_KONAMI |
ROM_K5 | Alias for ROM_KONAMI_SCC |
In your project_config.js, chose as target one of the above target type. You can specify the ROM size (in KB) in the ROMSize variable. Default value is 128 (KB). Any multiple value of the mapper's segment size is valid, but we recommend using powers of 2 values (64, 128, 256, etc.) to help emulators to autodetect the right mapper.
Some Build Tool configuration examples:
//-- 128 KB ASCII-8 mapped-ROM target = "ROM_ASCII8";
//-- 4 MB ASCII-16 mapped-ROM target = "ROM_ASCII16"; ROMSize = 4096;
//-- 512 KB Konami SCC mapped-ROM target = "ROM_KONAMI_SCC"; ROMSize = 512;
For more detail:
- Create a mapped ROM article.
- projects/samples/s_vgm sample for a concrete use case.
The Build tool deployment step places your final program in emul/rom/ folder.
Driver
Target format to create program that can be load to a given place in the memory space and been execute from there (so, like a driver).
Target | Description |
---|---|
RAW | Raw binary code and data to be compiled at a given address |
DRIVER | Alias for RAW |
s_drv sample showcase how to create this kind of program and s_dos2 sample showcase the use of this driver.
Basically, a driver is an independent project that start with it's own main() function. Can be:
void main(); u8 main(); void main(u8 argv); // argv is set by the main program when driver is invoked u8 main(u8 argv);
Build a driver generate a .BIN file. When you want to use it in another program, just load the driver where it have been build too, then use:
void CallDriver(u16 addr, u8 a);
For example, you can load the driver .BIN in memory at 0xE000 then call:
CallDriver(0xE000, 0);
Needed configuration (in project_config.js):
//-- Target program format (string) Target = "RAW"; //-- Overwrite code starting address (number). For example. 0xE0000 for a driver in RAM ForceCodeAddr = 0xE000; //-- Overwrite RAM starting address (number). For example. 0xE0000 for 8K RAM machine ForceRamAddr = 0xF000;
Notes:
- There is no check for driver's variables to verify they not override with main program variables, so be sure to set ForceRamAddr to a free memory space.
- Note also that to limit driver overhead, global variables are not initialized.
Library
Target format to create C library to be used in another program (can be code and/or data).
Target | Description |
---|---|
LIB | C library to be included in other project |
Build a C library generate a .LIB file.
Note that only the project configuration options, which concern the Compilation and Deployment steps, are used in the case of a library. Compilation will create the .LIB file, while Deployment will copy it into a /lib/ directory and add all the header files of the source codes that make it up.
When you want to use this library in another project, simply copy it there and add it to the AddLibs list in the project configuration.
Targets overview
Target | Selected at boot | Description | |||
---|---|---|---|---|---|
P0 | P1 | P2 | P3 | ||
ROM_8K | BIOS | Cart | RAM | RAM | 8 KB ROM in page 1 (4000h ~ 5FFFh) |
ROM_8K_P2 | BIOS | RAM | Cart | RAM | 8 KB ROM in page 2 (8000h ~ 9FFFh) |
ROM_16K | BIOS | Cart | RAM | RAM | 16 KB ROM in page 1 (4000h ~ 7FFFh) |
ROM_16K_P2 | BIOS | RAM | Cart | RAM | 16 KB ROM in page 2 (8000h ~ BFFFh) |
ROM_32K | BIOS | Cart | Cart | RAM | 32 KB ROM in page 1&2 (4000h ~ BFFFh) |
ROM_48K | BIOS | Cart | Cart | RAM | 48 KB ROM in page 0-2 (0000h ~ BFFFh) |
ROM_48K_ISR | Cart | Cart | Cart | RAM | 48 KB ROM in page 0-2 (0000h ~ BFFFh) with ISR replacement |
ROM_64K | BIOS | Cart | Cart | RAM | 64 KB ROM in page 0-3 (0000h ~ FFFFh) |
ROM_64K_ISR | Cart | Cart | Cart | RAM | 64 KB ROM in page 0-3 (0000h ~ FFFFh) with ISR replacement |
ROM_ASCII8 | BIOS | Cart | Cart | RAM | ASCII-8: 8KB segments for a total of 64 KB to 2 MB |
ROM_ASCII16 | BIOS | Cart | Cart | RAM | ASCII-16: 16KB segments for a total of 64 KB to 4 MB |
ROM_KONAMI | BIOS | Cart | Cart | RAM | Konami MegaROM (aka Konami4): 8 KB segments for a total of 64 KB to 2 MB |
ROM_KONAMI_SCC | BIOS | Cart | Cart | RAM | Konami MegaROM SCC (aka Konami5): 8 KB segments for a total of 64 KB to 2 MB |
ROM_NEO8 | Cart | Cart | Cart | RAM | NEO mapper: 8 KB segments for a total up to 32 MB |
ROM_NEO16 | Cart | Cart | Cart | RAM | NEO mapper: 16 KB segments for a total up to 64 MB |
ROM_YAMANOOTO | BIOS | Cart | Cart | RAM | Yamanooto: 8 KB segments for a total up to 8 MB |
ROM_ASCII16X | BIOS | Cart | Cart | RAM | ASCII16-X: 16 KB segments for a total up to 64 MB |
DOS1 | RAM | RAM | RAM | RAM | MSX-DOS 1 program (starting at 0100h) |
DOS2 | RAM | RAM | RAM | RAM | MSX-DOS 2 program (starting at 0100h) |
DOS0 | RAM | RAM | RAM | RAM | Direct program boot from disk (starting at 0100h) |
BIN_DISK | BIOS | BASIC | RAM | RAM | BASIC binary on disk (starting at 8000h) |
BIN_TAPE | BIOS | BASIC | RAM | RAM | BASIC binary on tape (starting at 8000h) |
BIN_USR | BIOS | BASIC | RAM | RAM | BASIC USR binary driver (starting at C000h) |
RAW | -- | -- | -- | -- | Raw binary code and data to be compiled at a given address |
LIB | -- | -- | -- | -- | C library to be included in other project |
Main
For ROM or Basic program, the main function prototype is:
void main();
For DOS1 target, this prototype can also be used to get command-line arguments (set DOSParseArg to allow arguments parsing):
void main(u8 argc, const c8** argv);
For DOS2 target, this prototype can also be used to return a value du MSX-DOS:
u8 main(); u8 main(u8 argc, const c8** argv);
- argc: Integer that contains the number of arguments present in argv. The argc parameter can be 0 if no parameter have been added to the command line.
- argv: Array of null-terminated strings representing command-line arguments entered by the user of the program.
- return: The return value can be catch by MSX-DOS 2 as an error code to know if program process succeeded or not.